Introduction to HTTP for frontend development
Auteur(s) de l'article
In this vast world of the web, every interaction, whether it's opening a web page, submitting a form or watching a video, is based on a protocol called HTTP (HyperText Transfer Protocol). In this article I'm going to share the basics that I think are important for frontend developers to know.
The role of HTTP is to act as a messenger between the client (the user's browser) and the server.
When you enter a URL or simply click on a link, you send an HTTP request. The server recognises (or not) the request and sends back a response with the content you requested.
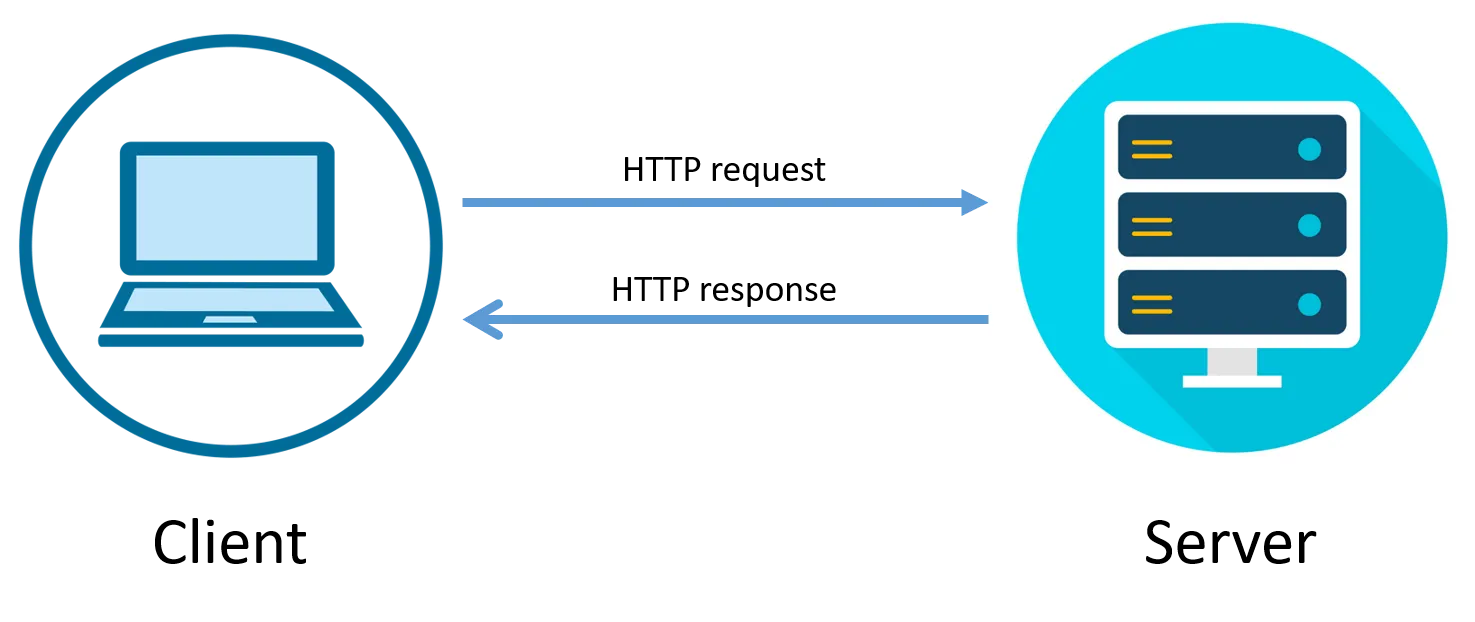
We could draw an analogy with a restaurant, for example. You (the browser/customer) place your order (HTTP request). The order is taken to the kitchen (the web server), the order is then prepared and brought back to you (HTTP response).
Why is it important for frontend developers to understand the HTTP protocol?
As a front-end developer, you frequently have to interact with services coming from the back-end. Knowing the HTTP protocol will enable you to communicate effectively with these services in order to process data in the most optimal way for your site.
You've probably already heard the term CORS, or you've already received a 404 error. It's in these cases that knowledge of the HTTP protocol helps you to understand what's happening to you.
It can also arouse your curiosity and enable you to develop the skills to use techniques such as lazy loading, caching, and so on. All this to offer better performance to your users.
Beyond GET and POST
HTTP verbs, often called HTTP methods, define the action we intend to perform on a given resource.
These methods offer much more than just the ability to retrieve web pages with
GET
or submit data with POST
.Here are some methods you might find useful (let's continue with culinary analogies):
GET
- Analogy: Getting milk from the fridge.
- Case study: Retrieving data. When you load a web page, your browser uses a
GET
request to retrieve the content.
POST
- Analogy: Adding a new bottle of soda to your fridge.
- Case in point: Submitting data. When you fill in a registration form on a website, the data you enter is sent to the server using a
POST
request.
PUT
- Analogy: Replace your out-of-date mayonnaise with a fresh new tube.
- Case in point: Updating existing data or creating it if it doesn't exist. If a user wants to update their profile information, a frontend application could use a query
PUT
.
PATCH
- Analogy: Add an extra slice of cheese to your sandwich.
- Case in point: Partially update a resource. For example, if a user only wants to change their e-mail address on a profile,
PATCH
allows this without affecting the other data.
DELETE
- Analogy: Compost your wilted salad.
- Case in point: Deleting a resource. If a user decides to delete their account, a
DELETE
request would be initiated.
You can find the full list anywhere online, for example on the mozilla doc.ㅤ
Using
GET
and POST
alone can result in unnecessary data transfers, leading to slower applications. On the other hand, the appropriate use of verbs such as PATCH
can optimise data transfer, saving time and bandwidth, which is a real plus for sustainable development.418 I'm a teapot
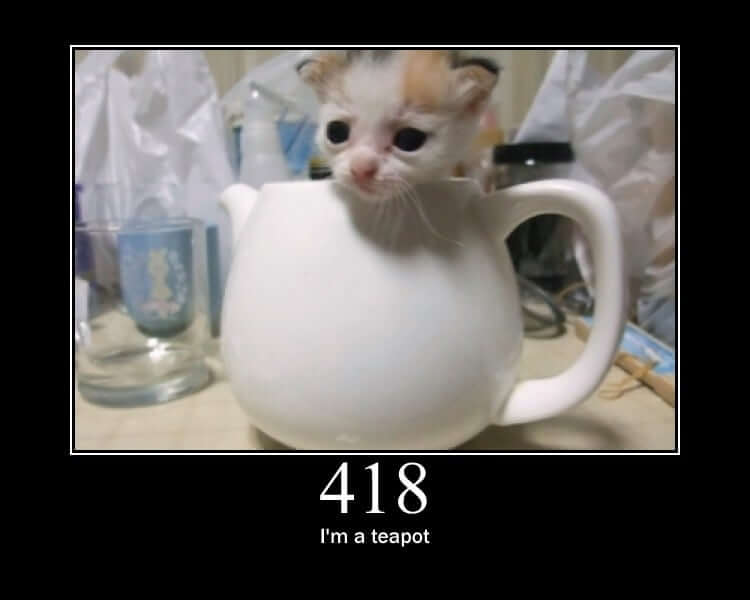
It's a good idea to know what a
Status Code
is, or at least what the different status categories represent. This will make debugging easier and may save you from acute headaches.HTTP statuses are grouped into five categories represented by the first digit of the code. Each category indicates the general result of the request. If you like cats, visit https://http.cat/ for some great examples.
1xx
(Informative) : These codes generally indicate a provisional response. The actual final response will follow the informative response.2xx
(Successful): This category indicates that the customer's request has been successfully received, understood and accepted.3xx
(Redirection): These codes indicate that further action is required to complete the request, often in the form of a redirect.4xx
(Customer errors): These codes indicate that the customer appears to have made an error. They are used when, for example, the request contains incorrect syntax or cannot be fulfilled.5xx
(Server errors): These codes indicate that the server has been unable to fulfill a valid request. The server itself is aware that it has an error or is unable to complete the request.
Headers
The postman has come to deliver your parcel ordered the day before from [insert your favourite e-commerce store beginning with the letter G].
Even before you open the box, the label stuck to the carton gives you valuable information: the sender, the destination, any special instructions, the market value, etc.
In the same way, HTTP headers provide crucial information about the request or response, indicating how it should be handled, what its content is, how it should be cached, who has authorisations.
Here is a non-exhaustive list of headers that I think are important to know:
Accept
Specifies the types of media that can be processed. This allows servers to adapt their responses to the client's capabilities. For example, an
Accept
header can indicate that the client accepts responses in JSON (application/json
) or XML (application/xml
) format.Accept: application/json
User-Agent
Identifies the browser, operating system and other characteristics of the browser. This allows the server to provide an optimised response based on the client's capabilities. Frontend developers can sometimes use this header to make browser/OS-specific adjustments.
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36
Authorization (with Bearer)
This header is crucial for secure requests. When a user is authenticated, the server generally expects an access token. The most common scheme is the "Bearer" scheme, used with JWT tokens.
Authorization: Bearer eyJhbGciOiJ-IUzI1NiIhssInR5cCI6IkpX-VCJ9.eyJzddWIiOqiIxMjM-0NTY3ODkwIdiwibmg-FtZSI6Ikpv-aG4gRG9lIiwi-aWF0IjoxNTsdfE2MjM5M-DIyfQ.SflKxwRJSMeKK-F2QT4fwpMeJf36POk-6ymjJV-adQssw5c
Content-Type
Indicates the media type of the request or response
body
. For requests, this informs the server about the format of the data sent, while for responses, it tells the client how to interpret the content.Content-Type: application/json
Cookie
This header contains the cookies that must be included in the request. Cookies are used, for example, to maintain the state of the session between the client and the server.
Cookie: sessionId=123456; userId=9876
Cache-Control
Specifies caching directives in requests and responses. Frontend developers can use this header to control the browser's caching behaviour.
Cache-Control: no-cache, no-store, must-revalidate
By understanding these few frequently used headers, frontend developers can improve request management, security and overall website efficiency.
Payloads
What is a payload?
In the context of HTTP, a payload refers to the data transmitted in a request or response. This data can take various forms, such as text, JSON, XML or even binary data. Since this has a direct impact on the data your applications send and receive, I think it's important to talk about it in this article.
Payload types
Here are some classic examples of the type of content used by us frontends:
Text-Based Payloads :
HTML
: The most common on the web, HTML contains the structure and content of a page.JSON
: Widely used for data, JSON is a lightweight, easily readable format that frontend developers often manipulate when interacting with APIs.
Binary Payloads :
Images
: Image data, such as JPEG or PNG files, are often transmitted as binary payloads in HTTP requests and responses.Fichiers
: When users download files, the binary payload is used to transmit the file data.
Structured Payloads :
XML
(eXtensible Markup Language) : Much less common than JSON, XML is another format for structuring data in a way that is both human-readable and machine-readable.
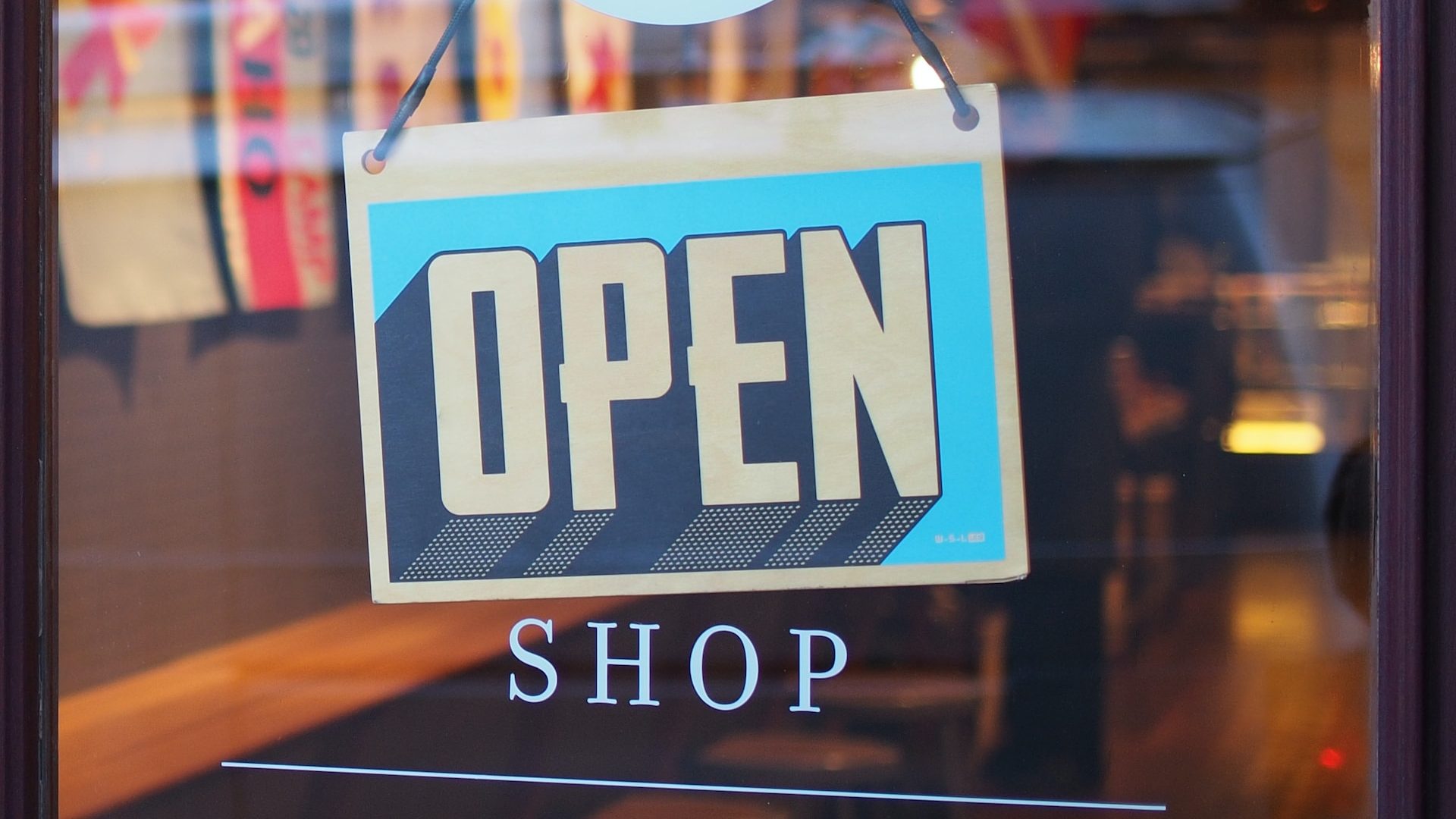
Let's take the example of an e-commerce site
Your aim is to implement a feature that allows users to add products to their basket without having to refresh the entire page.
Asynchronous basket updates:
Without an understanding of payloads, the frontend developer could struggle to communicate effectively with the backend when a user adds an item to their basket. Here, the payload would contain the product information (ID, name, quantity, etc.) that needs to be sent to the server.
Dynamic user interface updates:
Once the server processes the payload and updates the basket status, the response payload (for example in JSON format) becomes important. It needs to be interpreted to dynamically update the user interface. Thanks to this, its basket updated without having to reload the page.
Error handling :
If, for whatever reason, the server encounters a problem, the error payload becomes essential. Frontend devs can use this response to display a useful error message to the user.