We will explore the theory and then present some examples from real life.
But, as I always say, no explanation is complete without clarifying the benefits and challenges first.
What is it?
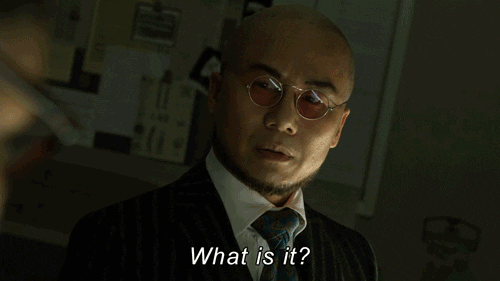
To make it short, refactoring is the art of increasing the maintainability of a piece of code.
“ There are two ways of constructing a software design. One way is to make it so simple that there are obviously no deficiencies. And the other way is to make it so complicated that there are no obvious deficiencies. ”C.A.R. Hoare
Actually, during your career you will always be refactoring code (yours or others).
“ On a standard 101-key keyboard, there are 100 keys that increase technical debt, and only one key that reduces it ”Josh Wulf
Of course, during a refactoring process you may find bugs. The moment that you start dealing with them, you've stopped refactoring and started bug fixing, which is another mindset.
I was speaking with a young developer the other day who was trying to convince me that refactoring a codebase makes it obviously faster.
It is a very common misconception that bad code automatically leads to performance leaks. The performance of your code is not the goal of a refactoring process - nor even what you should expect out of it.
When you are refactoring a part of your code, you should not change its behaviour, otherwise you are no longer refactoring.
Should you do it?
If an application that was written for a long time and remained then untouched is working as expected, if there's no conflict with any other system, if no features need to be added in the future, then it can be the ugliest code in the world: it doesn't need to be refactored.
You did not fix bugs, you did not make it faster, you did not add any new feature
So, you may ask me “why should I refactor then?”.
Well, the answer is quite simple. Well-structured code will make it so much easier to add new features and new capabilities in the future. And, it will make it easier to start analysing performance or overall improving the application.
“ It's called technical debt, because it's like taking out a loan. You can accomplish more today than you normally could, but you end up paying a higher cost later ”
How do we do it ?
For non-developer people, this part may be more tricky. Fly, you fools !
Bored of reading theory? Alright – me too – let’s dig into some real-life examples.
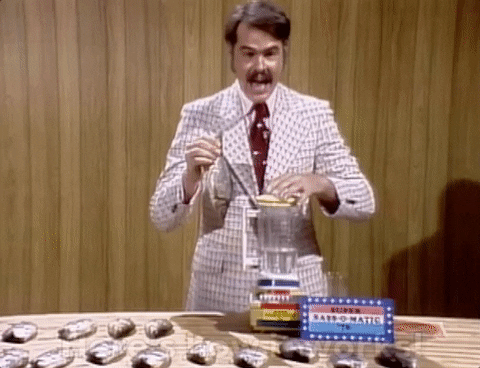
“ Always code as if the guy who ends up maintaining your code will be a violent psychopath who knows where you live. ”Martin Golding
The main reason is maybe pretty obvious, but let me say it out loud again: without unit tests, you will end up with broken functionalities that are nearly impossible to fix because you won't be able to figure out what's happening anymore.
So please, if you have to refactor something, first make sure it is covered by tests.
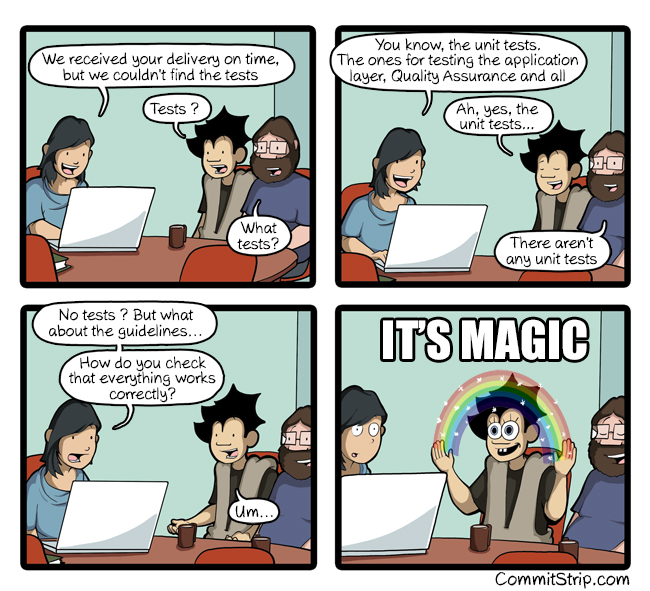
Conditional Guard Clauses (return early)
Assignments to Parameters
Very often, this occurs accidentally and leads to unfortunate effects. Even if parameters are usually passed by value (and not by reference), this coding quirk may be weird to those who are unaccustomed to it.
Replace Array with Object
Arrays
are an excellent tool for storing data and collections of a single type.But if you use an array like post office boxes, storing the username in box 1 and the user's address in box 14, you will someday be very unhappy that you did that way.
Object
over Array
, you will be able to apply properties assertions, I would suggest everybody to take a look at the ArrayAccess
implementations which is great to replace object-like Array
.Replace Magic Number with Symbolic Constant
Consolidate Conditional Expression
Use the language capabilities
Many of these features can save you a lot of effort. Take a look at the next examples and notice how it can be easy to achieve the same result by using the type
hinting
methodology.Inline Temp
What else ?
- Use a new array form
[ ]
instead of the oldarray()
- Use
===
operator instead of==
unless it is important to not check for thedataType
- Use prefix
is/has
with functions that return boolean ex:isAdmin($user)
,hasPermission($permission, $user)
- Organise class methods with public methods at the top
- Always apply the single responsibility concept to your classes
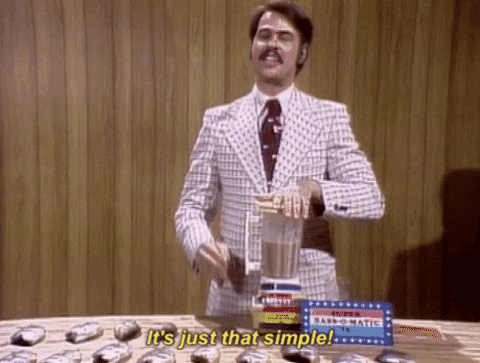
Sources
See on https://sourcemaking.com/refactoring
See on https://refactoring.guru/refactoring
See on https://medium.com/hackernoon/refactor-your-php...
See on https://medium.com/@sitapati/there-is-no-good-...
See on https://medium.com/@fionnachan/code-ref...
See on https://medium.com/@tdeniffel/brutal-refactorin...
See on https://medium.com/@sroze/refactoring-the...
See on https://hackernoon.com/refactori...
See on https://kotaku.com/the-except...